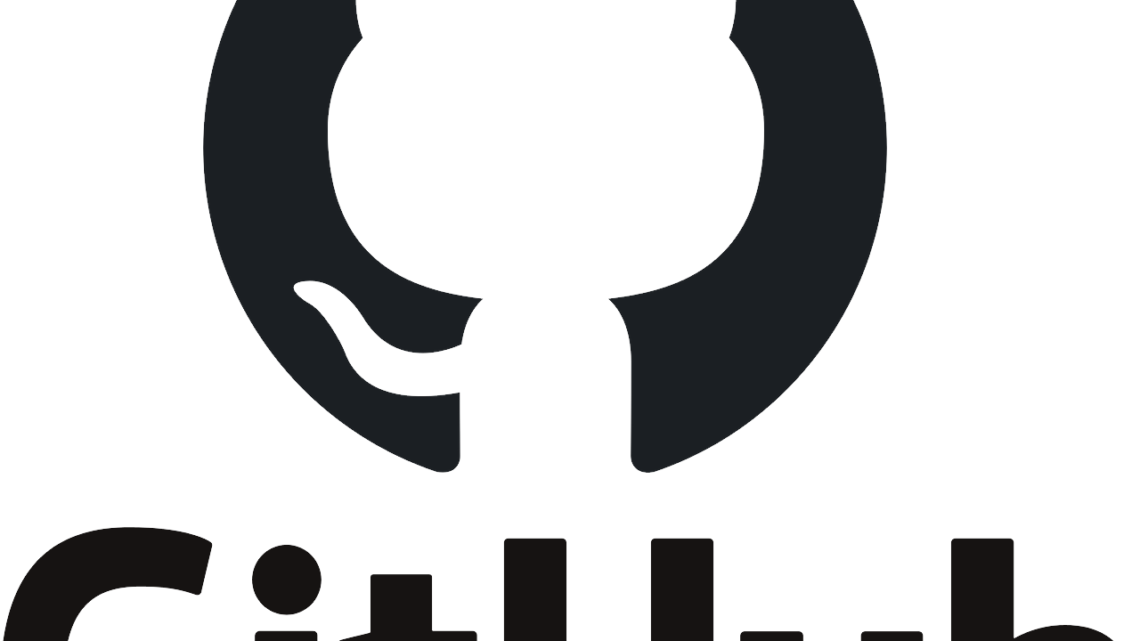
Ansible Playbook from Github Action
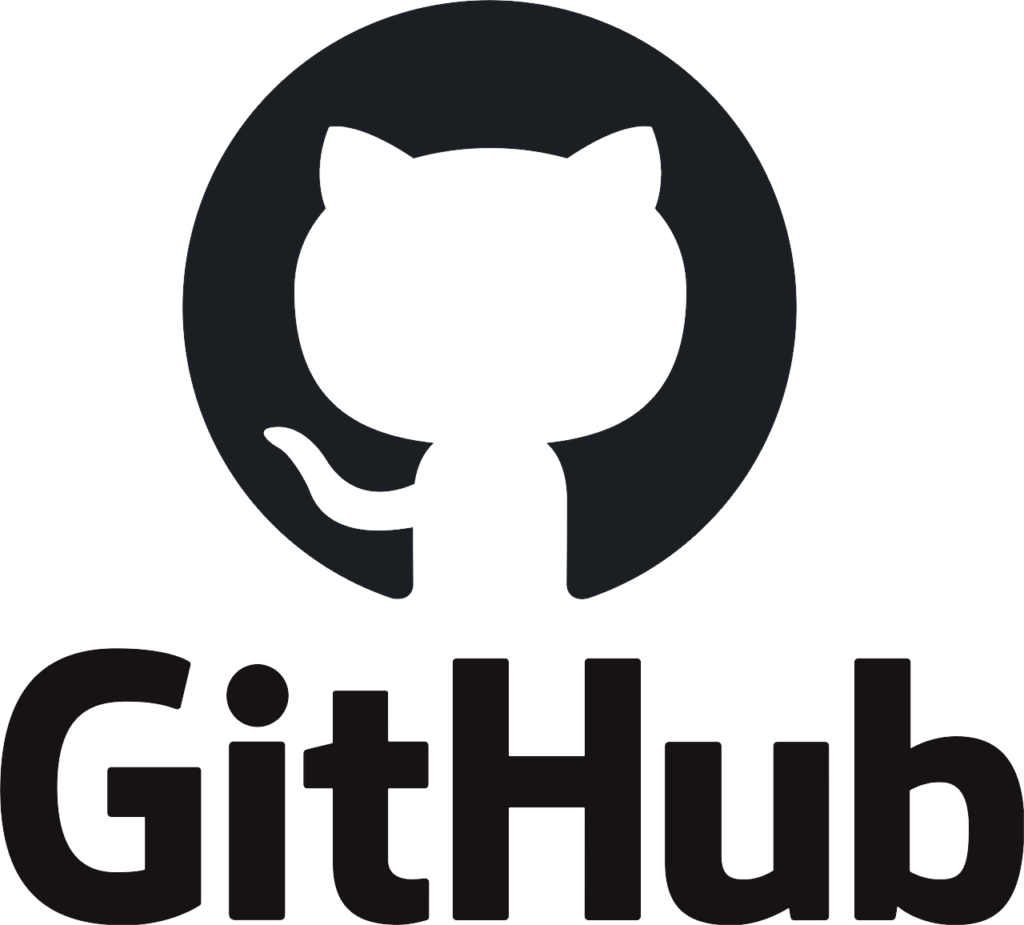
I’ve recently started a side project with Ansible and I wanted to automatise the Ansible playbook deployment with Github Action. Here is a tiny tutorial on how to use Github actions to run ansible playbooks to deploy stuff on Digital Ocean Droplets.
SSH Key
Before creating digital ocean droplets, you need to create a private ssh key.
ssh-keygen -f .ssh/id_digital_ocean
Then copy the content of the public key in your digital ocean account.
cat .ssh/id_digitalocean.pub
Go to your Digital Ocean account security page and add a new ssh-key.
You need to specify this key when you create a droplet. Here is the command to get the md5 checksum of the key used in droplet creation :
ssh-keygen -E md5 -l -f .ssh/id_digitalocean.pub
Digital Ocean Droplet
Digital Ocean Droplets are created with the digital_ocean_droplet
ansible module. You can use the digital ocean dynamic inventory script to retrieve droplet list : Just copy this file and the .ini in the inventory directory of your workspace.
The inventory script require to export the DO_API_KEY in environment variables.
To create a Digital Ocean API key, simply go to your console UI and click on API on the left menu, then generate a new token
Github Secrets
To use Ansible in Github Action, we need to create Github Secrets in the project. Go to your project settings, and in the secret menu add the following variables :
ANSIBLE_VAULT_PASSWORD
: The ansible vault password used to encrypt / decrypt secret variablesDO_API_KEY
: The Digital Ocean API KeyDO_SSH_KEY
: The private ssh key used by ansible to connect to droplets.
GitHub Action
Now it time for Action !
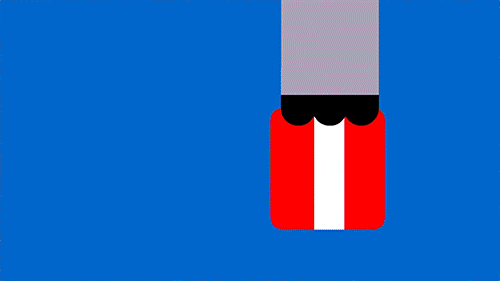
Github Action is like gitlab-ci : create a .github/workflows/deploy.yml
file in your git project ans specify the steps you want.
name: Ansible deploy
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v1
- name: Set up Python 3.7
uses: actions/setup-python@v1
with:
python-version: 3.7
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install ansible==2.9.2 requests
- name: set ansible config secrets
env:
ANSIBLE_VAULT_PASSWORD: ${{ secrets.ANSIBLE_VAULT_PASSWORD }}
DO_SSH_KEY: ${{ secrets.DO_SSH_KEY }}
run: |
echo "$ANSIBLE_VAULT_PASSWORD" > .ansible-vault-password
mkdir .ssh
echo "$DO_SSH_KEY" > .ssh/id_digitalocean
chmod 600 .ssh/id_digitalocean
- name: run exporters playbook
env:
DO_API_KEY: ${{ secrets.DO_API_KEY }}
run: |
ansible-playbook -i inventory play.yml
Then, when I git push to my repo, I can see my github action running
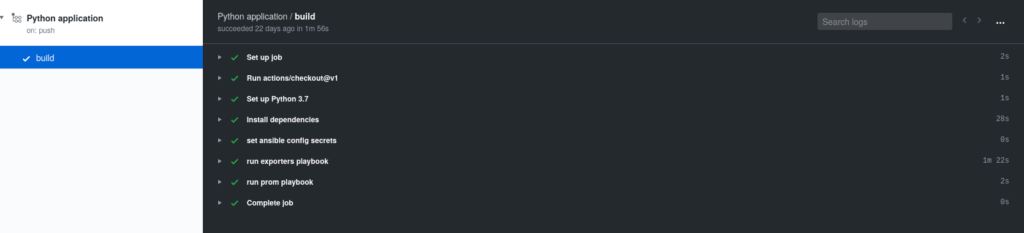
Enjoy !